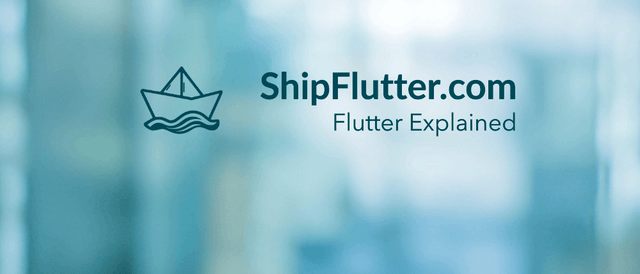
AnimatedBuilder Explained | Flutter for Web Developers
Understand how to use AnimatedBuilder as an Web Developer
What’s AnimatedBuilder?
The AnimatedBuilder class is a general-purpose widget in Flutter designed for building animations. It allows developers to create complex widgets that incorporate animations as part of a larger build function. Key properties include ‘animation’, which is the Listenable object that triggers rebuilds, and ‘builder’, a function called every time the animation notifies about a change.
Check the official documentation for more.
AnimatedBuilder explained for Web Developers
From a web developer’s perspective, the AnimatedBuilder class can be compared to React’s use of hooks for managing state and effects. Just like React components can re-render based on state changes, the AnimatedBuilder listens to changes in animations or other Listenable objects, allowing for efficient updates without needing to manage the animation state manually. This is similar to how frameworks like Angular and Vue handle reactive data binding.
Example Code
Here is an example of how to use AnimatedBuilder:
import 'package:flutter/material.dart';
class SpinningSquare extends StatelessWidget { @override Widget build(BuildContext context) { return AnimatedBuilder( animation: AnimationController( duration: const Duration(seconds: 2), vsync: this, )..repeat(), builder: (context, child) { return Transform.rotate( angle: animation.value * 2.0 * 3.14159, child: Container( width: 100, height: 100, color: Colors.green, ), ); }, ); }}
AnimatedBuilder Remarks
In conclusion, the AnimatedBuilder class is a powerful tool for Flutter developers, enabling efficient animation management and integration into complex widget trees. By understanding its properties and methods, web developers can leverage their existing knowledge of reactive programming to create dynamic and engaging user interfaces.
Bootstrap Your app with ShipFlutter
A fully customizable starter kit to seamlessly launch responsive Android, iOS, and Web apps with Flutter powered by Firebase and Vertex AI.