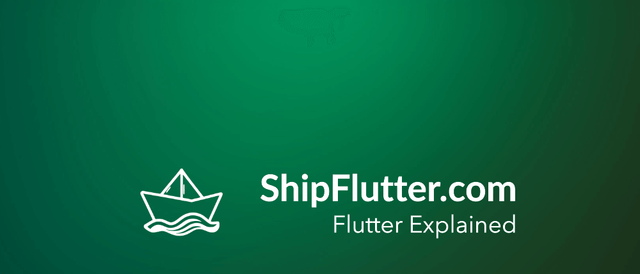
AnimationController Explained | Flutter for Android Developers
Understand how to use AnimationController as an Android Developer
What’s AnimationController?
The AnimationController class is a key component in Flutter for managing animations. It allows developers to control the playback of animations, including starting, stopping, and reversing them. Important methods include forward(), reverse(), and stop(), which facilitate the animation’s lifecycle.
Check the official documentation for more.
AnimationController explained for Android Developers
For Android developers, the AnimationController can be compared to the Animator class in Android, which also manages animations. Just like in Android, where you can start an animation and control its duration, the AnimationController provides similar functionality but is more integrated with Flutter’s reactive framework. The use of TickerProvider in Flutter is akin to using ValueAnimator in Android, where you need a mechanism to update the animation frames.
Example Code
Here is an example of how to use AnimationController:
class Foo extends StatefulWidget { const Foo({ super.key, required this.duration }); final Duration duration; @override State createState() => _FooState();}
class _FooState extends State<Foo> with SingleTickerProviderStateMixin { late AnimationController _controller;
@override void initState() { super.initState(); _controller = AnimationController( vsync: this, duration: widget.duration, ); }
@override void dispose() { _controller.dispose(); super.dispose(); }
@override Widget build(BuildContext context) { return Container(); // ... }}
AnimationController Remarks
In conclusion, the AnimationController class in Flutter provides a powerful and flexible way to manage animations, similar to the animation frameworks in Android. Understanding its methods and lifecycle is crucial for creating smooth and responsive animations in your Flutter applications.
Bootstrap Your app with ShipFlutter
A fully customizable starter kit to seamlessly launch responsive Android, iOS, and Web apps with Flutter powered by Firebase and Vertex AI.