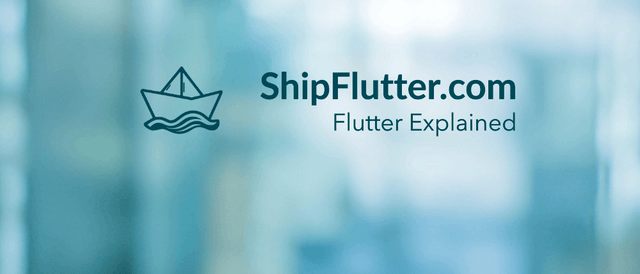
AnimationController Explained | Flutter for Web Developers
Understand how to use AnimationController as an Web Developer
What’s AnimationController?
The AnimationController class in Flutter is a powerful tool for managing animations. It allows developers to play animations forward or in reverse, stop them, and set specific values. Key methods include forward(), reverse(), stop(), and properties like value, duration, and status.
Check the official documentation for more.
AnimationController explained for Web Developers
From a web developer’s perspective, the AnimationController is similar to animation libraries in frameworks like React, Angular, or Vue, where you can control animations through state changes. However, Flutter’s AnimationController is more explicit, requiring a TickerProvider to manage frame updates, akin to using requestAnimationFrame in JavaScript.
Example Code
Here is an example of how to use AnimationController:
class Foo extends StatefulWidget { const Foo({ super.key, required this.duration }); final Duration duration; @override State createState() => _FooState();}
class _FooState extends State<Foo> with SingleTickerProviderStateMixin { late AnimationController _controller;
@override void initState() { super.initState(); _controller = AnimationController( vsync: this, duration: widget.duration, ); }
@override void dispose() { _controller.dispose(); super.dispose(); }
@override Widget build(BuildContext context) { return Container(); // ... }}
AnimationController Remarks
In conclusion, the AnimationController class is essential for creating smooth animations in Flutter. Understanding its lifecycle and how to manage it effectively will enhance your Flutter applications.
Bootstrap Your app with ShipFlutter
A fully customizable starter kit to seamlessly launch responsive Android, iOS, and Web apps with Flutter powered by Firebase and Vertex AI.