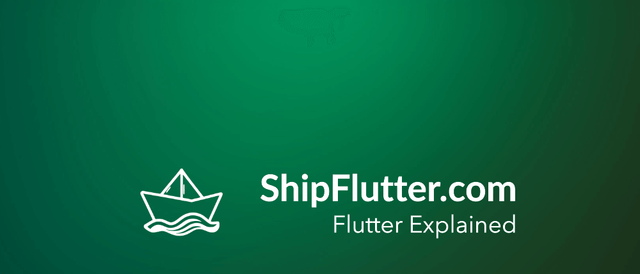
ChangeNotifier Explained | Flutter for Android Developers
Understand how to use ChangeNotifier as an Android Developer
What’s ChangeNotifier?
The ChangeNotifier class is a mixin that provides a change notification API for Flutter applications. It allows objects to notify listeners when changes occur, making it easier to manage state in applications. Key methods include addListener, removeListener, and notifyListeners, which facilitate the registration and notification of listeners.
Check the official documentation for more.
ChangeNotifier explained for Android Developers
For Android developers, the ChangeNotifier class can be compared to LiveData or MutableLiveData in Android’s architecture components. Just as LiveData allows UI components to observe changes in data, ChangeNotifier enables Flutter widgets to listen for changes in state. This is particularly useful in Flutter’s reactive framework, similar to how Jetpack Compose uses State and MutableState to manage UI state.
Example Code
Here is an example of how to use ChangeNotifier:
class Counter extends ChangeNotifier { int _count = 0;
int get count => _count;
void increment() { _count++; notifyListeners(); }}
void main() { final counter = Counter(); counter.addListener(() { print('Count updated: ${counter.count}'); }); counter.increment(); // Output: Count updated: 1}
ChangeNotifier Remarks
In conclusion, the ChangeNotifier class is a powerful tool for managing state in Flutter applications. By understanding its functionality and how it relates to familiar concepts in Android development, developers can effectively implement reactive programming patterns in their Flutter apps.
Bootstrap Your app with ShipFlutter
A fully customizable starter kit to seamlessly launch responsive Android, iOS, and Web apps with Flutter powered by Firebase and Vertex AI.