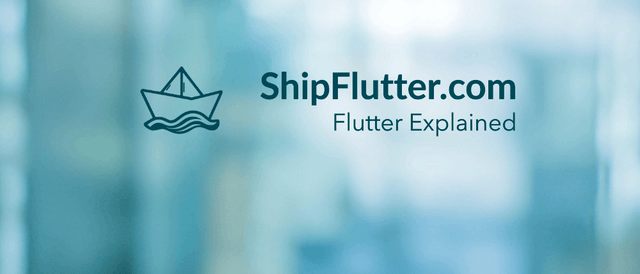
ChangeNotifier Explained | Flutter for Web Developers
Understand how to use ChangeNotifier as an Web Developer
What’s ChangeNotifier?
The ChangeNotifier class is a mixin in Flutter that provides a change notification API. It allows classes to notify listeners when changes occur. Important methods include addListener, removeListener, and notifyListeners, which manage listener registration and notification.
Check the official documentation for more.
ChangeNotifier explained for Web Developers
From a Web Developer’s perspective, ChangeNotifier is similar to state management solutions in frameworks like React (useState/useEffect), Angular (Services), and Vue (Vuex). It allows for reactive programming by notifying UI components when the underlying data changes, ensuring that only the necessary parts of the UI are updated.
Example Code
Here is an example of how to use ChangeNotifier:
class Counter extends ChangeNotifier { int _count = 0;
int get count => _count;
void increment() { _count++; notifyListeners(); }}
void main() { final counter = Counter(); counter.addListener(() { print('Count: ${counter.count}'); }); counter.increment(); // Output: Count: 1}
ChangeNotifier Remarks
ChangeNotifier is a powerful tool for state management in Flutter, allowing developers to create responsive applications that efficiently update the UI based on data changes.
Bootstrap Your app with ShipFlutter
A fully customizable starter kit to seamlessly launch responsive Android, iOS, and Web apps with Flutter powered by Firebase and Vertex AI.