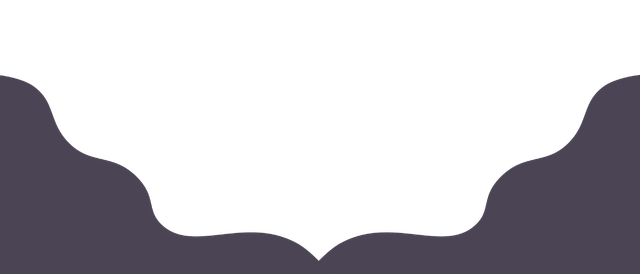
Firebase Emulator with a Physical Device | Flutter Explained
Go beyond the simulator to test your app.
Are you trying to connect your physical Android or iOS device to the Firebase emulator and getting this error?
[firebase_auth/unknown] An internal error has occurred. [ Failed to connect to /10.0.2.2:9099 ]
Are you wondering why it works with the simulator/emulator on your computer?
Well… your physical device is not in the same localhost network! (doh!)
Here is how you can enable your device to connect to the Firebase emulator:
- Configure the Firebase emulator to accept outside connecitons by adding this to your
firebase.json
:
"emulators": { "auth": { "host": "0.0.0.0", "port": 9099 }, "functions": { "host": "0.0.0.0", "port": 5001 }, "firestore": { "host": "0.0.0.0", "port": 8080 }, // ... others}
-
Find your machine local IP address:
- Windows: Open Command Prompt and type ipconfig. Look for the “IPv4 Address” under your network connection.
- macOS: Open System Preferences, go to Network, and select “details” on your active network connection. The IP address should be visible there.
- Linux: Open a terminal and type hostname -I, which will display the IP address of your machine.
-
Configure your app to use the emulator at the local IP address (this example uses Flutter but it’s similar for any other language):
if (kDebugMode) { // Run with flutter run --dart-define="localhost=true" // to enable localhost emulator instead of using the cloud. // Make sure the ports match the ones defined in firebase.json // https://firebase.google.com/docs/functions/local-emulator if (const bool.fromEnvironment("localhost")) { // Disable persistence for emulators to avoid inconsitent data store.settings = const Settings(persistenceEnabled: false);
// Get the IP address if any, otherwise use localhost const ip = String.fromEnvironment("ip", defaultValue: "127.0.0.1"); await auth.useAuthEmulator(ip, 9099); store.useFirestoreEmulator(ip, 8080); functions.useFunctionsEmulator(ip, 4000); await storage.useStorageEmulator(ip, 9199); }}
- Create a launch configuration to use localhost and/or IP address (vscode example):
{ "version": "0.2.0", "configurations": [ { "name": "Flutter app", "cwd": "app", "program": "lib/main.dart", "request": "launch", "type": "dart" }, { "name": "Flutter app - local", "cwd": "app", "program": "lib/main.dart", "request": "launch", "args": [ "--dart-define", "localhost=true", ], "type": "dart" }, { "name": "Flutter - local + physical", "cwd": "app", "program": "lib/main.dart", "request": "launch", "args": [ "--dart-define", "localhost=true", "--dart-define", "ip=192.168.86.26", ], "type": "dart" }, ]}
That’s it! your physical device should be able to connect to the network. The downside is that everytime the IP address changes your will have to update your launch configuration.
Bootstrap Your app with ShipFlutter
A fully customizable starter kit to seamlessly launch responsive Android, iOS, and Web apps with Flutter powered by Firebase and Vertex AI.