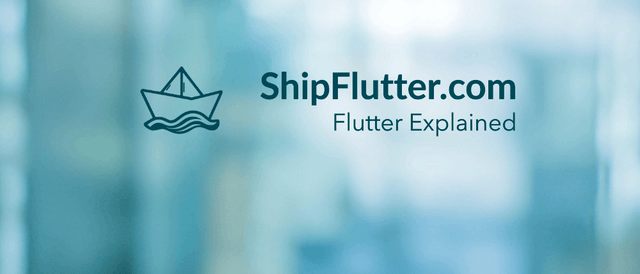
Hero Explained | Flutter for Web Developers
Understand how to use Hero as an Web Developer
What’s Hero?
The Hero class in Flutter is a widget that marks its child as a candidate for hero animations. It allows for smooth transitions between routes by animating a widget from one screen to another. Important properties include ‘tag’, which identifies the hero, and ‘child’, which is the widget that will animate.
Check the official documentation for more.
Hero explained for Web Developers
From a web developer’s perspective, the Hero class can be compared to animations in web frameworks like React or Vue, where components can transition smoothly between states. However, Flutter’s Hero animations are specifically designed for route transitions, making them unique in their implementation and usage.
Example Code
Here is an example of how to use Hero:
import 'package:flutter/material.dart';
class HeroExample extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('Hero Animation Example')), body: Center( child: GestureDetector( onTap: () { Navigator.push( context, MaterialPageRoute( builder: (context) => SecondPage(), ), ); }, child: Hero( tag: 'hero-tag', child: Container( width: 100, height: 100, color: Colors.blue, ), ), ), ), ); }}
class SecondPage extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('Second Page')), body: Center( child: Hero( tag: 'hero-tag', child: Container( width: 200, height: 200, color: Colors.blue, ), ), ), ); }}
Hero Remarks
The Hero class is a powerful tool for creating visually appealing transitions in Flutter applications. By understanding its properties and methods, web developers can leverage similar concepts from web frameworks to enhance user experience.
Bootstrap Your app with ShipFlutter
A fully customizable starter kit to seamlessly launch responsive Android, iOS, and Web apps with Flutter powered by Firebase and Vertex AI.