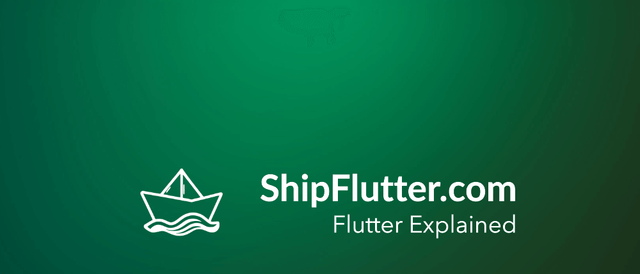
InheritedModel Explained | Flutter for Android Developers
Understand how to use InheritedModel as an Android Developer
What’s InheritedModel?
The InheritedModel class is an abstract class in Flutter that extends InheritedWidget. It is designed for models where dependents may only rely on specific aspects of the overall model. Unlike InheritedWidget, which rebuilds all dependents unconditionally, InheritedModel allows dependents to specify which aspect they depend on, leading to more efficient rebuilds.
Check the official documentation for more.
InheritedModel explained for Android Developers
For Android developers familiar with LiveData or ViewModel, the InheritedModel class serves a similar purpose in managing state and notifying UI components of changes. Just as LiveData allows observers to react to specific data changes, InheritedModel enables widgets to rebuild only when the relevant aspect of the model changes, optimizing performance.
Example Code
Here is an example of how to use InheritedModel:
class MyModel extends InheritedModel<String> { const MyModel({Key? key, required Widget child}) : super(key: key, child: child);
final int? a; final int? b;
@override bool updateShouldNotify(MyModel oldWidget) { return a != oldWidget.a || b != oldWidget.b; }
@override bool updateShouldNotifyDependent(MyModel oldWidget, Set<String> dependencies) { return (a != oldWidget.a && dependencies.contains('a')) || (b != oldWidget.b && dependencies.contains('b')); }
static MyModel? maybeOf(BuildContext context, [String? aspect]) { return InheritedModel.inheritFrom<MyModel>(context, aspect: aspect); }}
InheritedModel Remarks
The InheritedModel class is a powerful tool for Flutter developers, allowing for efficient state management and widget rebuilding. By understanding its functionality, developers can create more responsive and performant applications.
Bootstrap Your app with ShipFlutter
A fully customizable starter kit to seamlessly launch responsive Android, iOS, and Web apps with Flutter powered by Firebase and Vertex AI.