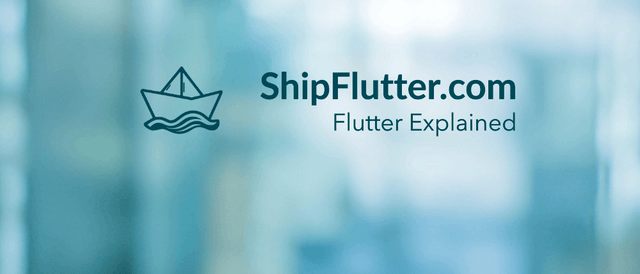
InheritedModel Explained | Flutter for Web Developers
Understand how to use InheritedModel as an Web Developer
What’s InheritedModel?
The InheritedModel class is an abstract class in Flutter that serves as a base for models whose dependents may only rely on specific aspects of the overall model. Unlike the InheritedWidget, which rebuilds all dependents unconditionally when the widget changes, the InheritedModel allows dependents to specify which aspect of the model they depend on, leading to more efficient rebuilds.
Check the official documentation for more.
InheritedModel explained for Web Developers
From a web developer’s perspective, the InheritedModel class can be compared to state management solutions in frameworks like React, Angular, or Vue. Just as these frameworks allow components to subscribe to specific pieces of state, the InheritedModel enables widgets to listen for changes in specific aspects of the model. This selective dependency can lead to performance improvements, as only the widgets that depend on the changed aspect will be rebuilt, similar to how React’s useContext can optimize re-renders.
Example Code
Here is an example of how to use InheritedModel:
class MyModel extends InheritedModel<String> { const MyModel({Key? key, required Widget child}) : super(key: key, child: child);
final int? a; final int? b;
@override bool updateShouldNotify(MyModel oldWidget) { return a != oldWidget.a || b != oldWidget.b; }
@override bool updateShouldNotifyDependent(MyModel oldWidget, Set<String> dependencies) { return (a != oldWidget.a && dependencies.contains('a')) || (b != oldWidget.b && dependencies.contains('b')); }
static MyModel? maybeOf(BuildContext context, [String? aspect]) { return InheritedModel.inheritFrom<MyModel>(context, aspect: aspect); }}
InheritedModel Remarks
For web developers transitioning to Flutter, understanding the InheritedModel class is crucial for efficient state management. By leveraging its selective rebuild capabilities, developers can create responsive and performant applications.
Bootstrap Your app with ShipFlutter
A fully customizable starter kit to seamlessly launch responsive Android, iOS, and Web apps with Flutter powered by Firebase and Vertex AI.