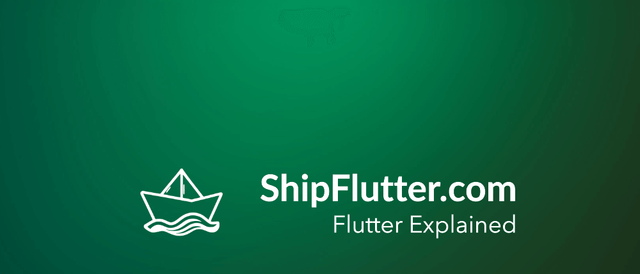
InheritedWidget Explained | Flutter for Android Developers
Understand how to use InheritedWidget as an Android Developer
What’s InheritedWidget?
The InheritedWidget class is a base class for widgets that efficiently propagate information down the widget tree. It allows widgets to access data from their ancestors in the widget tree. Key methods include ‘of’ and ‘maybeOf’, which help retrieve the nearest instance of a specific inherited widget.
Check the official documentation for more.
InheritedWidget explained for Android Developers
For Android developers, the InheritedWidget can be compared to the ViewModel in Android’s architecture components, where data is shared across different UI components. Just like LiveData allows UI components to observe changes, InheritedWidget enables widgets to rebuild when the inherited data changes, providing a reactive programming model similar to Jetpack Compose’s state management.
Example Code
Here is an example of how to use InheritedWidget:
class FrogColor extends InheritedWidget { const FrogColor({ super.key, required this.color, required super.child, }); final Color color;
static FrogColor? maybeOf(BuildContext context) { return context.dependOnInheritedWidgetOfExactType<FrogColor>(); }
static FrogColor of(BuildContext context) { final FrogColor? result = maybeOf(context); assert(result != null, 'No FrogColor found in context'); return result!; }
@override bool updateShouldNotify(FrogColor oldWidget) => color != oldWidget.color;}
InheritedWidget Remarks
In summary, the InheritedWidget class is a powerful tool in Flutter for managing state and data propagation. Understanding its functionality can greatly enhance an Android developer’s ability to create efficient and responsive applications in Flutter.
Bootstrap Your app with ShipFlutter
A fully customizable starter kit to seamlessly launch responsive Android, iOS, and Web apps with Flutter powered by Firebase and Vertex AI.