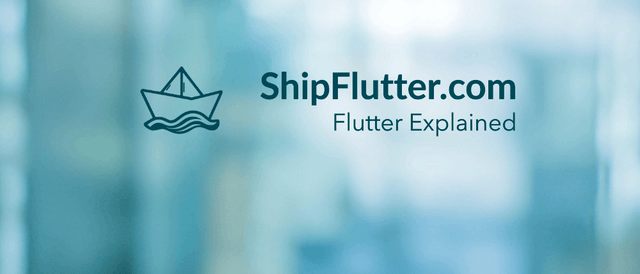
InheritedWidget Explained | Flutter for Web Developers
Understand how to use InheritedWidget as an Web Developer
What’s InheritedWidget?
The InheritedWidget class is a base class for widgets that efficiently propagate information down the widget tree. It allows widgets to access data from their ancestors in the widget tree. Key methods include ‘of’ and ‘maybeOf’, which help retrieve the nearest instance of a specific inherited widget.
Check the official documentation for more.
InheritedWidget explained for Web Developers
From a web developer’s perspective, InheritedWidget can be compared to context providers in frameworks like React, Angular, or Vue. Just as these frameworks allow components to access shared state or context, InheritedWidget enables Flutter widgets to access data from their parent widgets. This is particularly useful for managing state and ensuring that widgets rebuild when the inherited data changes.
Example Code
Here is an example of how to use InheritedWidget:
class FrogColor extends InheritedWidget { const FrogColor({ super.key, required this.color, required super.child, }); final Color color;
static FrogColor? maybeOf(BuildContext context) { return context.dependOnInheritedWidgetOfExactType<FrogColor>(); }
static FrogColor of(BuildContext context) { final FrogColor? result = maybeOf(context); assert(result != null, 'No FrogColor found in context'); return result!; }
@override bool updateShouldNotify(FrogColor oldWidget) => color != oldWidget.color;}
class MyPage extends StatelessWidget { const MyPage({super.key});
@override Widget build(BuildContext context) { return Scaffold( body: FrogColor( color: Colors.green, child: Builder( builder: (BuildContext innerContext) { return Text( 'Hello Frog', style: TextStyle(color: FrogColor.of(innerContext).color), ); }, ), ), ); }}
InheritedWidget Remarks
For web developers transitioning to Flutter, understanding the InheritedWidget is crucial for effective state management. It provides a powerful way to share data across the widget tree, similar to context providers in other frameworks.
Bootstrap Your app with ShipFlutter
A fully customizable starter kit to seamlessly launch responsive Android, iOS, and Web apps with Flutter powered by Firebase and Vertex AI.