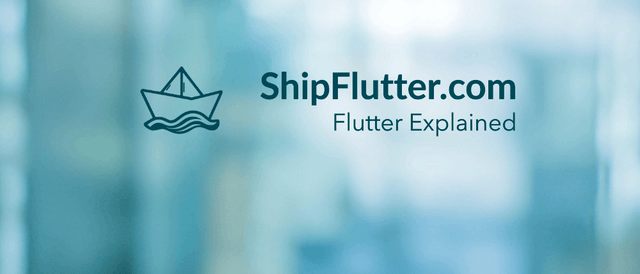
Notification Explained | Flutter for Web Developers
Understand how to use Notification as an Web Developer
What’s Notification?
The Notification class in Flutter is an abstract class that allows notifications to bubble up the widget tree. It is primarily used to communicate events from child widgets to their ancestors. Important methods include dispatch
, which sends the notification, and properties like runtimeType
that help identify the type of notification.
Check the official documentation for more.
Notification explained for Web Developers
From a web developer’s perspective, the Notification class can be compared to event handling in frameworks like React, Angular, or Vue. Just as these frameworks allow components to communicate through events, the Notification class enables widgets to send notifications up the widget tree, allowing parent widgets to respond to changes or events occurring in their children.
Example Code
Here is an example of how to use Notification:
import 'package:flutter/widgets.dart';
class MyNotification extends Notification { final String message; MyNotification(this.message);}
void main() { runApp(MyApp());}
class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return NotificationListener<MyNotification>( onNotification: (notification) { print(notification.message); return true; }, child: Center( child: ElevatedButton( onPressed: () { MyNotification('Hello from MyNotification!').dispatch(context); }, child: Text('Send Notification'), ), ), ); }}
Notification Remarks
The Notification class is a powerful tool for managing communication between widgets in Flutter. Understanding how to use it effectively can enhance your Flutter applications, making them more responsive and interactive.
Bootstrap Your app with ShipFlutter
A fully customizable starter kit to seamlessly launch responsive Android, iOS, and Web apps with Flutter powered by Firebase and Vertex AI.