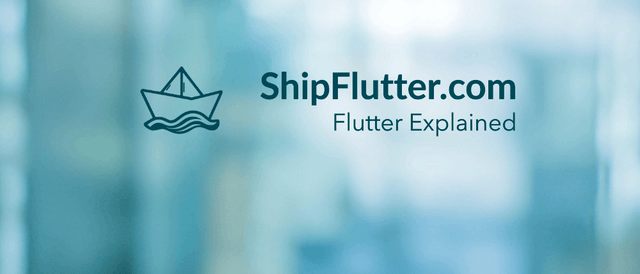
StatefulWidgetState Explained | Flutter for Web Developers
Understand how to use StatefulWidgetState as an Web Developer
What’s StatefulWidgetState?
The State class in Flutter is an abstract class that holds the logic and internal state for a StatefulWidget.
Check the official documentation for more.
StatefulWidgetState explained for Web Developers
State is information that can be read synchronously when the widget is built and might change during the widget’s lifetime. The widget implementer must ensure that the State is notified of changes using the setState method. This is somewhat similar to how state management works in Web APIs like React, Angular, and Vue, where components re-render based on state changes.
Example Code
Here is an example of how to use StatefulWidgetState:
class MyWidget extends StatefulWidget { @override MyWidgetState createState() => MyWidgetState();}
class MyWidgetState extends State<MyWidget> { int counter = 0;
void incrementCounter() { setState(() { counter++; }); }
@override Widget build(BuildContext context) { return Text('Counter: $counter'); }}
StatefulWidgetState Remarks
Understanding the State class and its lifecycle is crucial for managing state effectively in Flutter applications.
Bootstrap Your app with ShipFlutter
A fully customizable starter kit to seamlessly launch responsive Android, iOS, and Web apps with Flutter powered by Firebase and Vertex AI.