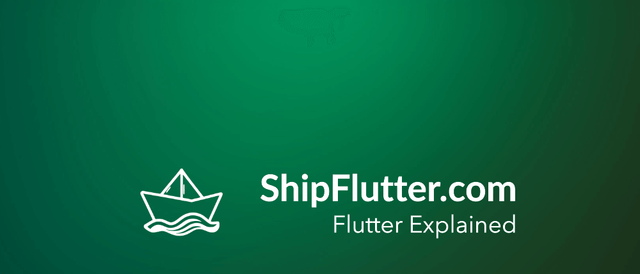
TickerProviderStateMixin Explained | Flutter for Android Developers
Understand how to use TickerProviderStateMixin as an Android Developer
What’s TickerProviderStateMixin?
The TickerProviderStateMixin class is a mixin that provides a Ticker to its child classes. It is primarily used in Flutter to create animations that require a Ticker, which is a mechanism that calls a callback at regular intervals.
Check the official documentation for more.
TickerProviderStateMixin explained for Android Developers
For Android developers, the TickerProviderStateMixin can be compared to using a Handler or a Timer in Android to perform actions at regular intervals. In Jetpack Compose, similar functionality can be achieved using the LaunchedEffect or rememberCoroutineScope to manage animations and state updates. The TickerProviderStateMixin simplifies the process of creating animations by providing a built-in mechanism to manage the timing of updates.
Example Code
Here is an example of how to use TickerProviderStateMixin:
class MyTickerProviderStateMixin extends StatefulWidget { @override _MyTickerProviderStateMixinState createState() => _MyTickerProviderStateMixinState();}
class _MyTickerProviderStateMixinState extends State<MyTickerProviderStateMixin> with TickerProviderStateMixin { late Ticker _ticker;
@override void initState() { super.initState(); _ticker = createTicker((elapsed) { // Update your animation here })..start(); }
@override void dispose() { _ticker.dispose(); super.dispose(); }
@override Widget build(BuildContext context) { return Container(); // Your widget here }}
TickerProviderStateMixin Remarks
In conclusion, the TickerProviderStateMixin is a powerful tool for Flutter developers looking to create smooth animations. By understanding its functionality and how it compares to familiar Android concepts, developers can easily integrate it into their Flutter applications.
Bootstrap Your app with ShipFlutter
A fully customizable starter kit to seamlessly launch responsive Android, iOS, and Web apps with Flutter powered by Firebase and Vertex AI.