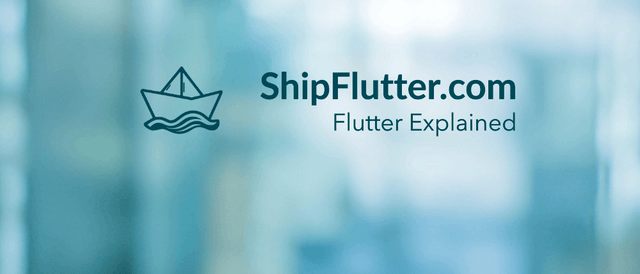
TickerProviderStateMixin Explained | Flutter for Web Developers
Understand how to use TickerProviderStateMixin as an Web Developer
What’s TickerProviderStateMixin?
The TickerProviderStateMixin class is a mixin that provides a Ticker to its child classes. It is primarily used in Flutter to create animations that require a continuous stream of ticks. Important methods include createTicker, which creates a Ticker that calls a callback on each tick, and dispose, which cleans up the Ticker when it is no longer needed.
Check the official documentation for more.
TickerProviderStateMixin explained for Web Developers
From a Web Developer’s perspective, the TickerProviderStateMixin can be compared to the concept of requestAnimationFrame in web APIs like React, Angular, or Vue. Just as requestAnimationFrame allows developers to create smooth animations by synchronizing updates with the browser’s refresh rate, TickerProviderStateMixin provides a way to create animations in Flutter by providing a continuous stream of ticks that can be used to update the UI.
Example Code
Here is an example of how to use TickerProviderStateMixin:
class MyTickerApp extends StatefulWidget {\n @override\n _MyTickerAppState createState() => _MyTickerAppState();\n}\n\nclass _MyTickerAppState extends State<MyTickerApp> with TickerProviderStateMixin {\n late Ticker _ticker;\n\n @override\n void initState() {\n super.initState();\n _ticker = createTicker((elapsed) {\n // Update your animation here\n });\n _ticker.start();\n }\n\n @override\n void dispose() {\n _ticker.dispose();\n super.dispose();\n }\n\n @override\n Widget build(BuildContext context) {\n return Container(); // Your widget here\n }\n}
TickerProviderStateMixin Remarks
In conclusion, the TickerProviderStateMixin is an essential tool for Flutter developers looking to implement animations. By understanding its functionality and how it relates to familiar web concepts, web developers can easily adapt to creating dynamic UIs in Flutter.
Bootstrap Your app with ShipFlutter
A fully customizable starter kit to seamlessly launch responsive Android, iOS, and Web apps with Flutter powered by Firebase and Vertex AI.